ChatViewList
Streamlined Chat List Integration: Easily Add a Message List to Your Dapp with Built-in Pagination
This component streamlines the process of displaying a paginated list of messages within your user interface. All you need to do is provide a chat ID, and the component handles the rest, presenting you with a comprehensive view of all messages. Additionally, it conveniently includes chat meta information at the top of the component for a complete chat experience.
Usage
- react
import { useContext } from "react";
import styled from "styled-components";
import { ChatViewList } from "@pushprotocol/uiweb";
const ChatViewListTest = () => {
return (
<ChatViewListCard>
<ChatViewList
chatId="196f58cbe07c7eb5716d939e0a3be1f15b22b2334d5179c601566600016860ac"
limit={10}
/>
</ChatViewListCard>
);
};
export default ChatViewListTest;
const ChatViewListCard = styled.div`
height: 40vh;
background: black;
overflow: auto;
overflow-x: hidden;
`;
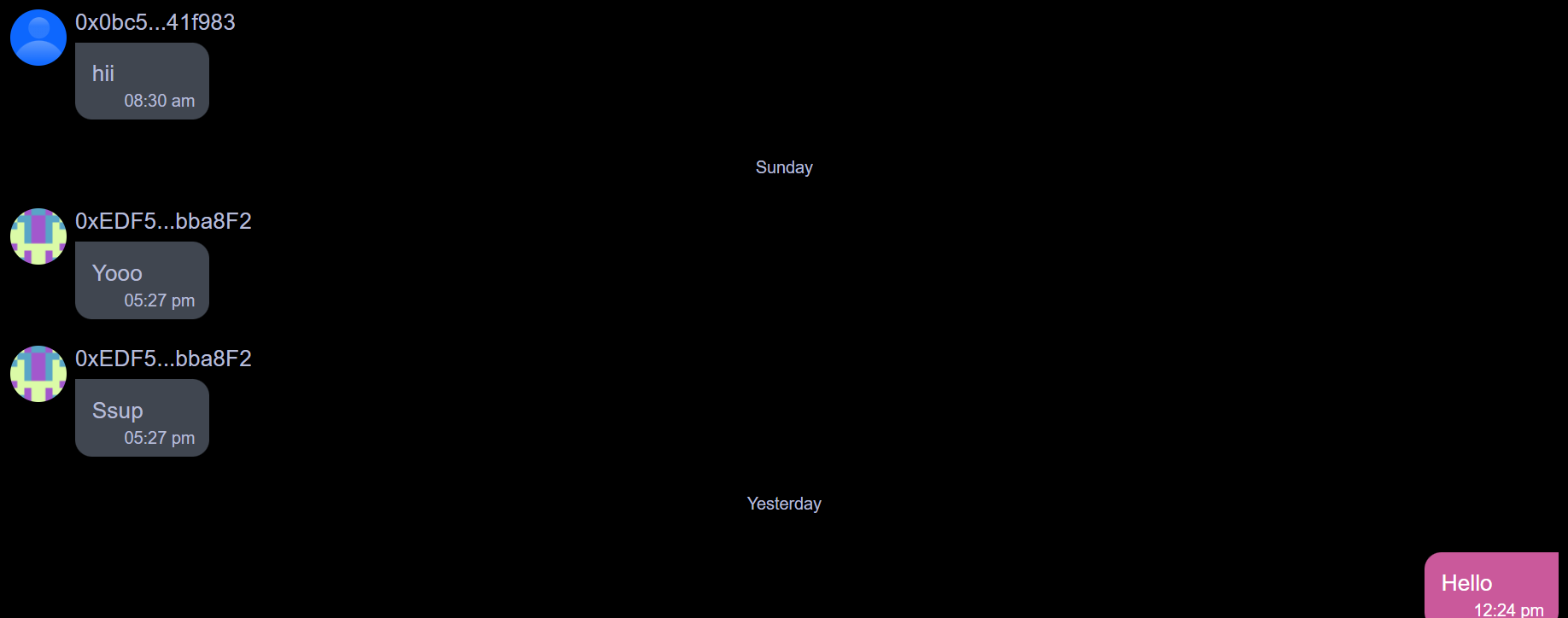
Customization parameters
Param | Type | Default | Remarks |
---|---|---|---|
chatId | string | - | Chat id for a particular chat |
limit | number | - | Number of messages fetched in each paginated api call,default value is 10 |
chatFilterList | Array<string> | - | Array of cid's of messages that needs to be excluded from chatViewList |
Note: Parameters
in this style
are mandatory.
Note: Refer ChatUIProvider for details on its paramters.
Live playground
customPropMinimized = 'true'; // DO NOT FORGET TO IMPORT LIBRARIES // NOT NEEDED HERE SINCE PLAYGROUND IMPORTS INTERNALLY // import { ChatUIProvider, ChatViewList } from @pushprotocol/uiweb; function App(props) { const [signer, setSigner] = useState(null); const connectWallet = async () => { // Demo only supports MetaMask (or other browser based wallets) and gets provider that injects as window.ethereum into each page const provider = new ethers.providers.Web3Provider(window.ethereum); // Get provider await provider.send('eth_requestAccounts', []); // Grabbing signer from provider const signer = provider.getSigner(); // store signer setSigner(signer); }; const disconnectWallet = async () => { setSigner(null); }; const buttonStyle = { padding: '10px 20px', backgroundColor: '#dd44b9', color: '#FFF', border: 'none', borderRadius: '5px', cursor: 'pointer', marginTop: '20px', }; return ( <> <h2>Interact with ChatViewList by changing the chatId.</h2> <ChatUIProvider signer={signer}> <label> For this demo, You will need Metamask (or equivalent browser injected wallet), you will also need to sign a transaction to see the notifications. Connect wallet for better usage of the ChatViewList component. </label> <p /> <button style={buttonStyle} onClick={signer ? disconnectWallet : connectWallet} > {signer ? 'Disconnect wallet' : 'Connect Wallet'} </button> <div style={{ height: '75vh', margin: '20px auto', overflow: 'hidden scroll', }} > <ChatViewList chatId='0x99A08ac6254dcf7ccc37CeC662aeba8eFA666666' /> </div> </ChatUIProvider> </> ); }
LIVE PREVIEW