CreateGroupModal
Effortless Modal Integration: Add a modal to create group effortlessly with CreateGroupModal.
Creating groups becomes a straightforward task with this modal component, completely eliminating the need to concern yourself with its functionality. By simply passing an onClose
method for the modal, you can effortlessly integrate it into your application. This versatility allows you to create both gated and non-gated groups without any hassle.
Usage
- react
import { CreateGroupModal } from "@pushprotocol/uiweb";
const CreateGroupModalTest = () => {
return (
<div>
<CreateGroupModal onClose={()=>{console.log('closes the modal')}}/>
</div>
);
};
export default CreateGroupModalTest;
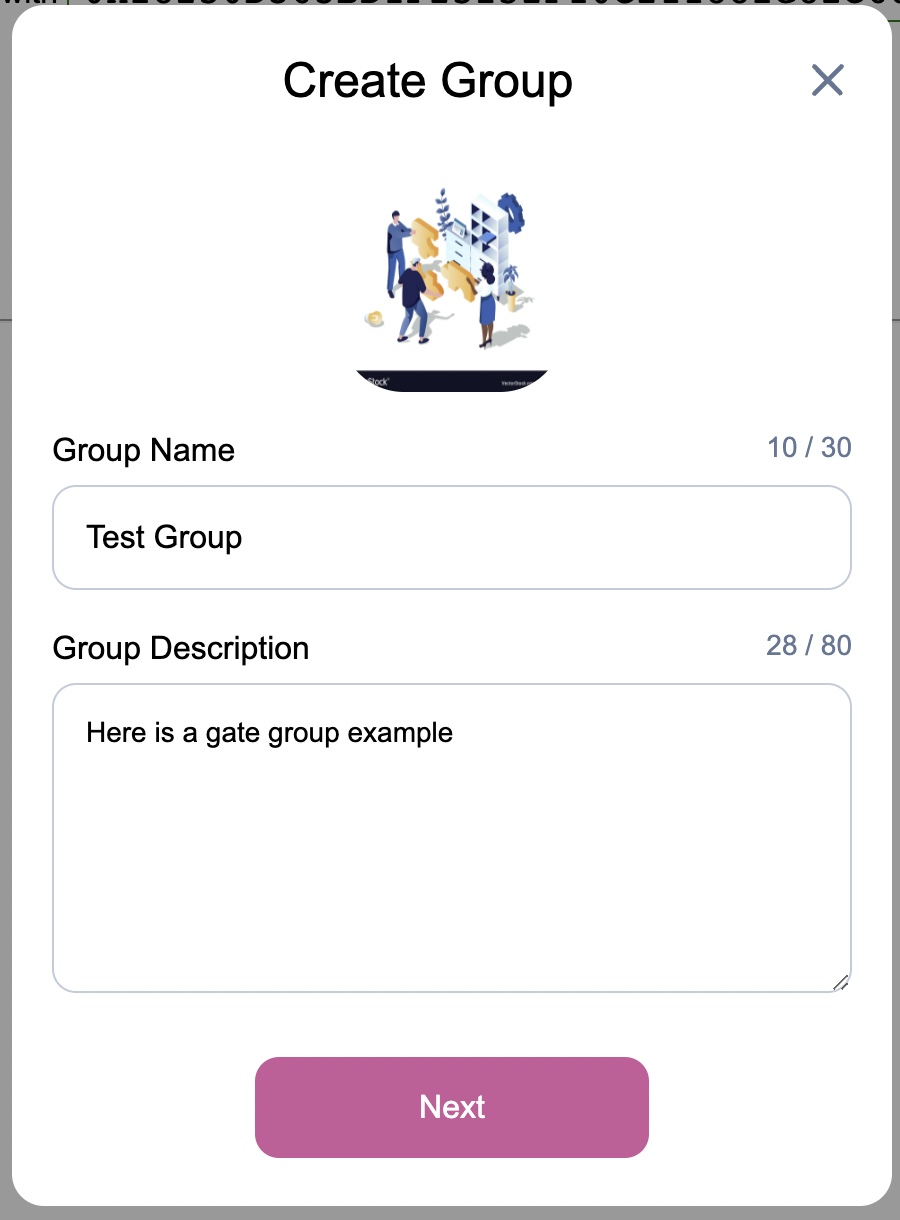
Customization parameters
Param | Type | Default | Remarks |
---|---|---|---|
onClose | function | - | Function to perfom any task on clicking the cancel or cross button on the modal |
modalBackground | ModalBackgroundType | - | Default value is "OVERLAY" , decides the create group modal background, possible values are "OVERLAY" | "BLUR" | "TRANSPARENT" |
modalPositionType | ModalPositionType | - | Default value is "GLOBAL" , decides the create group modal position, it can be either relative to immediate parent(RELATIVE) or the entire screen(GLOBAL), possible values are "RELATIVE" | "GLOBAL" |
Note: Parameters
in this style
are mandatory.
Note: Refer ChatUIProvider for details on its paramters.
Push CreateGroupModal Component live playground
customPropMinimized = 'true'; // DO NOT FORGET TO IMPORT LIBRARIES // NOT NEEDED HERE SINCE PLAYGROUND IMPORTS INTERNALLY // import { ChatUIProvider, CreateGroupModal,MODAL_POSITION_TYPE } from @pushprotocol/uiweb; function App(props) { const [signer, setSigner] = useState(null); const connectWallet = async () => { // Demo only supports MetaMask (or other browser based wallets) and gets provider that injects as window.ethereum into each page const provider = new ethers.providers.Web3Provider(window.ethereum); // Get provider await provider.send('eth_requestAccounts', []); // Grabbing signer from provider const signer = provider.getSigner(); // store signer setSigner(signer); }; const disconnectWallet = async () => { setSigner(null); }; const buttonStyle = { padding: '10px 20px', backgroundColor: '#dd44b9', color: '#FFF', border: 'none', borderRadius: '5px', cursor: 'pointer', marginTop: '20px', }; return ( <> <h2></h2> <label> For this demo, You will need Metamask (or equivalent browser injected wallet), you will also need to sign a transaction to see the notifications. Connect wallet for better usage of the CreateGroupModal component. </label> <p /> <button style={buttonStyle} onClick={signer ? disconnectWallet : connectWallet} > {signer ? 'Disconnect wallet' : 'Connect Wallet'} </button> <ChatUIProvider signer={signer}> <div style={{ height: '75vh', margin: '20px auto', position: 'relative' }} > <CreateGroupModal onClose={() => { console.log('closes the modal'); }} modalPositionType={MODAL_POSITION_TYPE.RELATIVE} /> </div> </ChatUIProvider> </> ); }
LIVE PREVIEW